Using JavaScript To Add JavaScript to a PDF Part 2
Using JavaScript instead of the Acrobat user interface can automate the process of adding scripts to PDFs
In Using JavaScript To Add JavaScript to a PDF Part 1 I outlined how to add document level scripts, page action scripts, and document action scripts using JavaScript. In this article I will outline how to use JavaScript to add scripts to form fields. The setAction method for form fields has two mandatory input parameters:
cTrigger (A string that sets the trigger for the action).
cScript (The JavaScript to be executed).
Take the Acrobat Pro/JavaScript eLearning Course Acrobat Like a Pro
Triggers
The following cTrigger parameters can be used to execute the script:
MouseUp (After the mouse button is pressed down and released back up)
MouseDown (After as the mouse button is pressed down)
MouseEnter (As soon as the mouse cursor enters the field area)
MouseExit (As soon as the mouse cursor exits the field area)
OnFocus (When the field receives the focus, either by clicking inside the field with the mouse, or tabbing into the field from another field)
OnBlur (When the field loses the focus, either by tabbing out of the field, or clicking the mouse anywhere else inside the program, including toolbars and white space on the page.).
NOTE: OnBlur also commits a field value after it is keyed in, so if you’re waiting for a calculation to occur after entering a number in a field, you must first “blur” by tabbing out of the field or clicking the mouse somewhere else. For dropdown fields and combo box fields, there’s a check box in the options tab of the field properties "Commit selected value immidiately". Checking this box commits the selected value immediately upon selection without having to blur out of the field. This option is good for calculations and other actions that are dependent on the selection.
Keystroke (When the keyboard buttons are pressed (the user starts typing in the field))
Validate (When the value of the field changes)
Calculate (When the value of ANY field in the form changes)
Format (When the field value is committed.
Example 1
Suppose you have a button field named myButton and you want to add a Mouse Up action that pops up an alert that says "You just clicked this button." Running the following script in the console will accomplish that:
this.getField("myButton").setAction('MouseUp','app.alert("You just clicked this button.")');
Example 2
Suppose you have three fields: "Total", "Subtotal 1", and "Subtotal 2". You want the Total field to have a calculation script that adds the values of Subtotal 1 and Subtotal 2. This can be accomplished by running the following script in the console:
this.getField("Total").setAction('Calculate','event.value='+
'this.getField("Subtotal 1").value + '+
'this.getField("Subtotal 2").value;')
These examples use the JavaScript console to add the scripts to the fields. That's good for practicing and testing. A more practical use is to automate field creation for several PDFs and add scripts to them using the Execute JavaScript command in the Action Wizard.
Custom Formats
The custom format options that are built into Acrobat Pro form fields often contain both a keystroke script to validate each keystroke and reject those that do not comply with the format, and a custom format script, to format the committed value. The problem is that these scripts are hidden.
The format in the field properties in the image above shows the way the field is formatted for comma separated numbers with two decimal places, but does not show the format and keystroke scripts that are executed when interacting with this field, and there is no script to read the format like there is with other field properties like fill color, alignment, text size, and text font, etc. So how do we obtain the scripts?
Obtaining The Format and Keystrokes Scripts Contained in Field Formats
The Preflight tool in Acrobat Pro contains an option to "Browse Internal PDF Structure". You will find the format and keystroke scripts inside that structure.
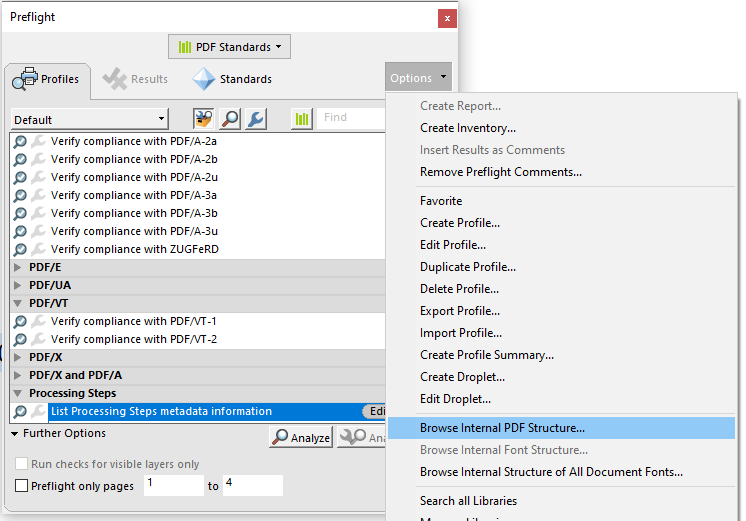
Before you do this it is highly recommended that you add a form field to a PDF that does not contain any form fields, then format the one-and-only field the way you want it before obtaining its scripts. This will make it much easier to find what you are looking for in an ever-expanding menu tree. You'll see what I mean as I outline the process in screenshots for a PDF containing only one form field called Total and formatted like the format screenshot above.
The format and keystroke scripts are inside the red rectangles in the image above. All the black triangles are tree expansions required to get to them. You can see how a form with multiple fields would make it difficult to find what you are looking for.
TIP: You can't copy and paste from the window pictured above. With the window open and the tree expanded, open Notepad so you can see the scripts while typing them out. Use the Notepad file to copy and paste from, then save it for future reference.
If you run the following script in the console it will format your Total field like the format screenshot above:
this.getField("Total").setAction('Format','AFNumber_Format(2,0,0,0,"",true)');
this.getField("Total").setAction('Keystroke','AFNumber_Keystroke(2,0,0,0,"",true)');
If you are curious, the comma separated list inside the brackets of AFNumber_Format and AFNumber_Keystroke are input parameters, in order:
nDec (number of decimal places)
sepStyle (0 = comma separated, 1 = no separator)
negStyle (0 = minus sign, 1 = red text, 2 = parenthesis, 3 = parenthesis and red text)
currStyle (Not used. Enter 0)
strCurrency (currency Symbol, eg. "$" or "£")
bCurrencyPrepend (currency symbol position: true=beginning, false=end - eg. 500$)
Another example
this.getField("Date").setAction('Format','AFDate_FormatEx("mm/dd/yyyy")');
this.getField("Date").setAction('Format','AFDate_KeystrokeEx("mm/dd/yyyy")');
If you have any question about this article, or suggestions for future articles, please leave them in the comment section below. See you next time…