The Acrobat Pro user interface (UI) has a tool for cropping PDF pages which can by accessed a few different ways, among them:
Press Shift + Ctrl + T on the keyboard (Windows Operating System)
Open the pages panel of the PDF, right-click a page and select Crop Pages.
Search Crop in the Tools tab and select Crop Page.
Similar to cutting a rectangle out of a physical paper page with scissors and discarding the excess, cropping a PDF page allows you to crop a rectangle out of an electronic page so you only see the cutout. The difference is that the excess is not discarded. While not visible on the screen, it remains in electronic format, and can be retrieved by reverse cropping.
Learn JavaScript For Acrobat Pro. Take the online course.
If your zoom is set to fit the width of the page, and you crop a portion of the page, the zoom will adjust to maintain the "fit width" magnification, making your page content appear much larger on the screen. If the page has form fields that have been cropped out you'll be able to see those fields by reducing the zoom (magnification). The fields will appear to be floating in space, as they will be in the grey area outside the visible page. You won’t be able to see other PDF content like text and images. If you open the cropping dialog by pressing Shift + Ctrl + T you will be able to see the other content in the dialog page view. The intent of this paragraph is to demonstrate that content is not lost when a page is cropped.
A consistent theme in this Substack is that almost everything that can be done with the Acrobat Pro UI can also been done with JavaScript, which is key in automating PDF tasks.
Cropping Pages Using JavaScript
The doc.setPageBoxes method takes four input parameters:
cBox - The type of box (either Art, Bleed, Trim, or Crop which were discussed in the PDF Page Coordinates article). Crop will be use throughout this article.
nStart - The 0-based starting page of the range of pages that will be operated on.
nEnd - The 0-based ending page of the range of pages that will be operated on.
rBox - An array of four numbers representing the box coordinates (clockwise from left: Left, Top, Right, Bottom - see PDF Page Coordinates for more details).
An 8.5" x 11" page has crop box coordinates of 0, 792, 612, 0. The left edge is always 0 and bottom is always 0. At 72 points per inch, 792 is 11 inches (bottom to top) and 612 is 8.5 inches (left to right).
Demonstration
You can follow along with this exercise by downloading and operating on this file. I created an 8.5" x 11" yellow page with a red rectangle with borders that are three inches from each side.
To crop this page around the red rectangle you can either do the math calculations for the crop box coordinates, or build them into the script and let the JavaScript engine do them for you. The page has crop box coordinates of 0, 792, 612, 0 but assume this is not known. First read the crop box coordinates using the doc.getPageBox method, then enter them into your calculation like this, running the following scrip in the JavaScript console:
var cb = this.getPageBox("Crop", 0);
this.setPageBoxes("Crop",0,0,[cb[0]+72*3, cb[1]-72*3, cb[2]-72*3, cb[3]+72*3]);
cb[0] (the left side) and cb[3] (the bottom) will always be zero so this part of the code could have been omitted, in which case the first and last indices of the array would both be 72*3, or three inches (at 72 points per inch). The script with the math calculations already completed and the page coordinates known is:
this.setPageBoxes("Crop", 0, 0, [216, 576, 396, 216]);
The left side of the new rectangle is three inches from the left edge of the page so three inches (216 points) are added to the left side. The top of the new rectangle is three inches from the top edge of the page so three inches are subtracted from the top edge. The right side of the new rectangle is three inches from the right edge of the page so three inches are subtracted from the right edge. And finally, the bottom side of the new rectangle is three inches from the bottom edge of the page, so three inches are added to the bottom edge.
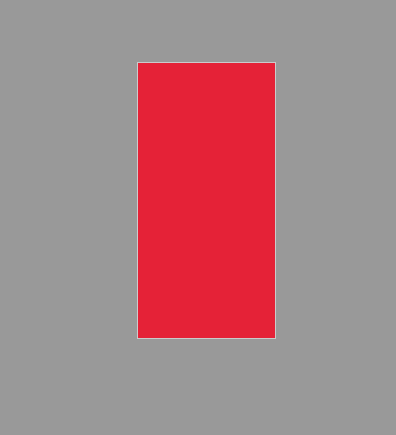
The Wrong Way To Reverse The Crop
The major use I have for cropping and then reversing is to significantly cut down the time it takes a script to extract words from a specific area of the page by first changing the crop box to that area, extracting all the words on the (new) page, then putting the page back the way it was by reversing the crop. If you think you can simply set the crop box back to 0, 792, 612, 0, you would be wrong. You see, everything is based on the 0/0 intersection of the left and bottom corner of the page. That means the crop box of the new page is [0, 360, 180, 0], not [216, 576, 396, 216].
If you’re doing the exercise run the following script in the console:
this.getPageBox("Crop",0);
The new top and right edges are based on the zero left and bottom. If you change the top to 792 it will add 11 inches from the NEW bottom edge. If you change the right edge to 612 it will add 8.5 inches from the NEW left side, moving the page up from bottom left corner of the red rectangle in this example. Here’s the result:
Try it. Run the following script in the console:
this.setPageBoxes("Crop", 0, 0, [0, 792, 612, 0]);
The Right Way To Reverse The Crop
The crop box must be extended outward on each side by subtracting what was added and adding what was subtracted.
In most cases the crop box will not be as clean and easy as this demonstration, with the same distance from every edge, and nice whole numbers. Usually you would obtain the crop box array by creating a field in the location you want to crop, then reading the rectangle coordinates (rect property) of the field. These are the same order as the page box coordinates, clockwise from the left: left, top, right, bottom. For example, if you created a field called Text1 exactly on top of the red rectangle in original file and ran the following script in the console it would return the crop box coordinates that were calculated (216, 576, 396, 216):
this.getField("Text1").rect;
When calculating the points from the page edges for the crop, simply assign variables that will be used in the reverse crop:
/* The rect array of the field, or the new crop box */
var rc=[216, 576, 396, 216];
/* The crop box page array */
var cb=this.getPageBox("Crop",0);
/* The reverse crop array */
var rc2=[cb[0]-rc[0],cb[1]-rc[3],cb[2]-rc[0],cb[3]-rc[3]];
Now crop the page:
this.setPageBoxes("Crop",0,0,rc);
Now read the new crop box coordinates (This step is not necessary in the process. It’s for demonstration purposes only):
this.getPageBox("Crop", 0);
Now reverse the crop:
this.setPageBoxes("Crop", 0, 0, rc2);
The Easy Way To Reverse Crop
In PDF Page Coordinates I stated that the Art, Bleed, Crop, and Trim page boxes usually have the same coordinates. All coordinates are relative to 0/0 bottom left corner of the crop box. If the Trim box was the same as the crop box prior to cropping, its coordinates will change when the page is cropped and left and bottom coordinates should go negative by the amount that was cropped. The following scrip can be run in the console to determine whether the Trim and Crop boxes are equal for each page:
for(var i=0; i<this.numPages; i++)
{
console.println("Page "+ i +": " +
(this.getPageBox("Crop",0).toString() == this.getPageBox("Trim",0).toString()))
}
The script above will return either true or false for each page, signifying whether the crop box is equal to the trim box. To obtain the rc2 variable from the previous example you would simply use the trim box coordinates after cropping. Here’s the script for this method:
this.setPageBoxes("Crop",0,0,[216, 576, 396, 216]);//crop
this.setPageBoxes("Crop",0,0,this.getPageBox("Trim",0));//reverse crop
To test that the trim box is correct after running the first line of code above (page is cropped), run this portion of the second line and it should return -216, 576, 396, -216:
this.getPageBox("Trim",0);
Which leads to…
The Easiest Way To Reverse Crop
Simply make the left and bottom of the new crop box array negative, and subtract the bottom of the crop from the top of the page, and the left of the crop from the right of the page:
/* The rect array of the field, or the new crop box */
var rc=[216, 576, 396, 216];
/* The crop box page array */
var cb=this.getPageBox("Crop",0);
/*Top and right of the array*/
var top=cb[1]-rc[3];
var right=cb[2]-rc[0];
/* The reverse crop array */
var rc2=[-rc[0],top,right,-rc[3]];
/*Now crop the page*/
this.setPageBoxes("Crop",0,0,rc);
/*Now reverse Crop*/
this.setPageBoxes("Crop",0,0,rc2);
In my next post I will demonstrate how to use crop/reverse to extract words from a specific area of the page. See you then.
If you find value in these articles, please help me grow my readership by sharing this article with others who might also find value here.